Matplotlib
- Matplotlib可幫助我們繪製圖形,例如曲線圖長條圖等。使用時須先import matplotlib.pyplot這個模組。
- import matplotlib並簡稱plt以方便程式撰寫。
- 使用plt.plot()方法來繪製圖形如下。其中參數可為list, tuple, array, Series等皆可。
- plt.plot()方法的格式可為如下兩類:
- plot([x], y, [fmt], data=None, **kwargs)
- plot([x], y, [fmt], [x2], y2, [fmt2], ..., **kwargs)
- x為x軸資料,y為y軸資料,fmt為format(顏色形狀等),由以上兩格式可看出x與format是optional,e.g.:。
- 上例中先建立10個元素的array x,再建立10個元素的Series y,然後繪製,'bo'的意思是藍色圓點。
- 上例若是沒有給format('bo'),則會defaultly繪製折線圖。
- 顏色的format有許多選擇,e.g:
- b: blue
- g: green
- r: red
- c: cyan
- m: magenta
- y: yellow
- b: black
- w: white
- #rrggbb: 16進位法方式顯示,此時無法直接與形狀合用。須用關鍵字。
- 形狀的format亦有許多選擇,e.g.:
- '-' or 'solid
- '--' or 'dashed'
- '-.' or 'dashdot'
- ':' or dotted
- 'None' or '' or ' '
- '.'
- ','
- 'o'
- 'v'
- '^'
- '<'
- '>'
- '1'
- '2'
- '3'
- '4'
- '8'
- 's'
- 'p'
- '*'
- 'h'
- 'H'
- '+'
- 'x'
- 'X'
- 'D'
- 'd'
- '|'
- '_'
- r"$\alpha$"
- r"$\beta$"
- r"$\gamma$"
- r"$\sigma$"
- r"$\infty$"
- r"$\spadesuit$"
- r"$\heartsuit$"
- r"$\diamondsuit$"
- r"$\clubsuit$"
- r"$\bigodot$"
- r"$\bigotimes$"
- r"$\bigoplus$"
- r"$\imath$"
- r"$\bowtie$"
- r"$\bigtriangleup$"
- r"$\bigtriangledown$"
- r"$\oslash$"
- r"$\ast$"
- r"$\times$"
- r"$\circ$"
- r"$\bullet$"
- r"$\star$"
- r"$+$"
- r"$\Theta$"
- r"$\Xi$"
- r"$\Phi$"
- r"$\$$"
- r"$\#$"
- r"$\%$"
- r"$\S$"
- Plot的其他參數: 以下為部分參數
- color: color = "#ff00ff"。
- dash_capstyle: 'butt', 'round','projecting'。
- dash_joinstyle: 'miter', 'round','bevel'。
- dashes: dashes(on, off)。e.g. dashes(5,5)。
- drawstyle: 'default', 'steps', 'steps-pre', 'steps-mid', 'steps-post'。
- linestyle or ls: 'solid', 'dashed', 'dashdot', 'dotted', (offset, on-off-dash-seq) e.g. (10,(5,5,10,2)), '-', '--', '-.', ':', 'None','',' '。
- fillstyle: fill the marker。'full', 'left', 'right', 'bottom', 'top', 'none'。
- linewidth or lw: linewidth = 5。
- marker: marker = r"$\beta$"。
- alpha: alpha=0.5。
- markeredgecolor or mec: mec = 'blue'。
- markeredgewidth or mew: marker edge width,e.g. mew = 1.5。
- markerfacecoloralt or mfcalt: mfcalt='pink'。
- markerfacecolor or mfc: marker face color,e.g. mfc = "green"。
- markersize or ms: ms = 10。
- markevery: 每間隔幾個設置marker。'None', 'int', (a,b), slice, list/array, float。
- snap: 繪於格子點。snap=True(None)。
- solid_capstyle: 'butt', 'round','projecting'。
- solid_joinstyle: 'miter', 'round', 'bevel'。
- Example:
pyplot1.py
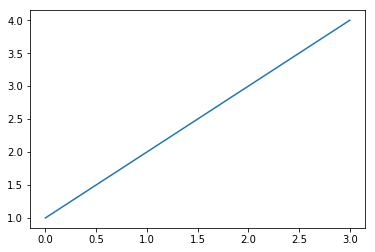
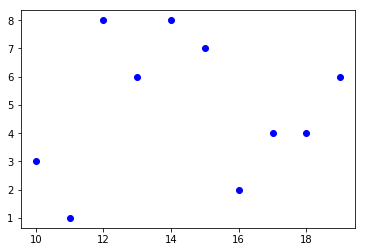
關於線段(linestyle):
關於點(Marker):
其他特殊點(使用屬性marker, e.g. marker = r"$\alpha$"):
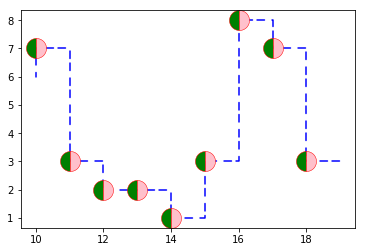
繪製多個圖型
- 只要將要繪製的圖型資料一一加入即可。
- plt.plot(x1,y1,'bo-',x2,y2,'r+--')會繪製兩條不同型式顏色的曲線。若是沒有加上'-'與'--',則只有點。
- 也可以分別繪製兩筆資料,若是要強調點跟線的不同,可將點跟線使用不同顏色表示,e.g.
pyplot2.py
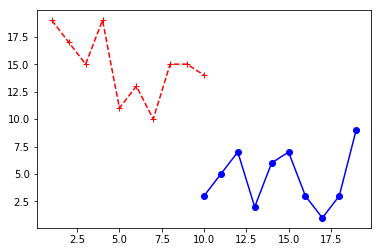
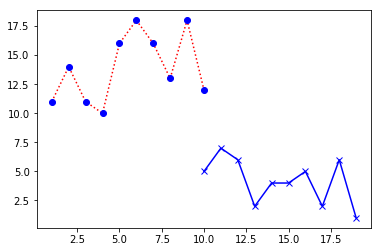
使用data繪製
- 若是有labeled的資料,例如dict,可以不抓取x,y,直接繪製。
- 使用plot([x], y, [fmt], data=None, **kwargs)其中的data。
- plt.plot('x1','y1','bx-',data=dic1)中第一個參數'x1'為dict中的第一筆資料名稱,'y1'為第二筆資料名稱,這兩筆資料分別為x,y的值。
- Pandas中的資料結構Series與DataFrame都支援plot()方法,直接使用Series.plot()或DataFrame.plot()即可。
- plot()中一樣有許多參數可以使用來修飾圖型,在console輸入到左括號時會出現關鍵字提示,也可以使用help(s.plot)查詢,其中多個參數會在後面介紹。
- 若要使用df中第一個Series為x,第二個Series為y來繪圖,可以直接取出x與y然後使用plt.plot()來繪圖,其實若不嫌麻煩,都可以將資料取出,然後都使用plt.plot()來繪製即可。
pyplot3.py
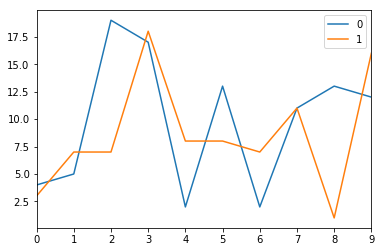
圖型的修飾
- 除了圖型顏色與線條,以下形式可以強化圖型的內容表示:
- plt.figure(figsize=(8,6))可設定圖型大小,但要放在其他設定之前。plt.figure()的部份其他屬性如下:
- figure(num=None, figsize=None, dpi=None, facecolor=None, edgecolor=None, frameon=True, FigureClass=
, clear=False, **kwargs) - 使用e.g. facecolor='#123456'與edgecolor='#abcdef'來改變背景顏色。
- dpi : integer, optional, default: None解析度,圖型大小跟著改變。
- plt.title()可建立圖型的title。
- fontdict: dictionary including fontsize, fontweight, verticalalignment(top, center, baseline), horizontalalignment(left, center, right)
- loc: title location, loc='left','center','right'。
- plt.xlabel()與plt.ylabel()可設定x,y軸的label。
- color: color='g'
- fontsize: fontsize = 15
- fontname: fontname = 'Times New Roman'
- plt.axis()用來設定axis的範圍。
- plt.grid(b=None, which='major', axis='both', **kwargs)。
- **kwargs可以使用line的參數,e.g. color="brown"。
- plt.tick_params(axis='both', **kwargs):改變tick的型態。
- plt.text(x, y, s, fontdict=None, withdash=False, **kwargs):可顯示文字於圖型。
- 在(x,y)位置顯示s文字。
- fontdict: 文字的性質,e.g. color='green'
- 若要顯示數學符號,可參考這個網頁
- plt.minorticks_on() vs plt.minorticks_off():開啟關閉minor ticks。
- plt.legend():顯示圖例。
- 定義在plot()之後。
- loc定義其位置,由0到10分別對應best, upper right, upper left, lower left, lower right, right,center left, center right, lower center, upper center, center。使用數字或文字皆可,亦即loc=1相等於loc='upper right'。可使用loc=0讓程式自己自動找最佳位置。
pyplot4.py
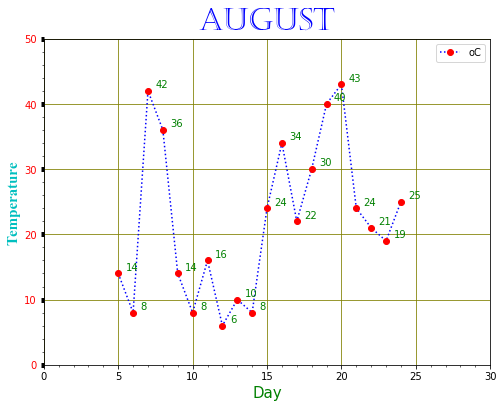
日期時間
- 如果某一軸是時間,因為時間需要較多文字顯示,顯示時可能會混雜在一起,例如:
- 上圖可見x軸的日期互相交錯在一起,難以辨識。可以嘗試以下方式:
pyplot5.py
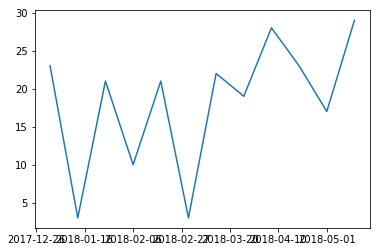
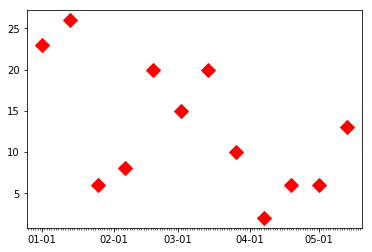
繪製數學函數
- 數學函數繪製
- 將x軸的tick顯示為pi,加上以下這一行。
- 繪製其他函數線段,並加上文字。
- 可以進一步使用以下方式改變軸的位置,在原來的code下加上以下程式碼。
- 使用plt.gca()方法取得圖型脊柱(spines),共有四邊。
- 將上方(top)與右邊(right)脊柱設定為不可見或是無顏色,e.g.set_visible(False) or set_color('none')
- 移動左邊(left)與下方(bottom)的spines至預定位置,使用data關鍵字,位置為0,表示以資料為中心,若修改數字則會移動至其他位置。(將data改為outword將由原位置開始計算移動)
- 使用ax.xaxis.set_ticks_position('bottom')方式先取得xaxis,然後設定其ticks位置。
pyplot6.py
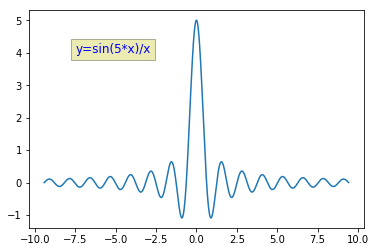
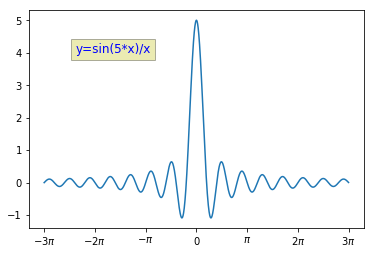
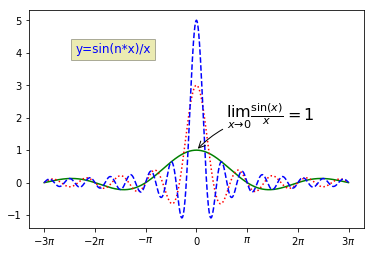
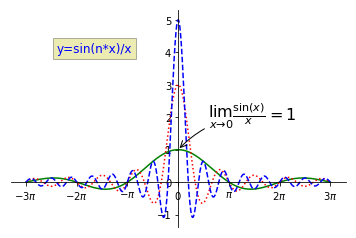
Multiple Figures & Axes
- 之前提過可以在一個圖表內繪製多個圖型,若是要繪製多個圖表並列做比較用途,可使用subplot。
- subplot(nrows, ncols, index, **kwargs): plt.subplot(211)中的211代表的意思是2個row,1個column,其中的第1個圖。212就代表2個row,1個column,其中的第2個圖。
- 依此類推,若是圖型為左右並列,則使用(121)與(122),若是排列成2x2形式,則使用(221)(222)(223)(224),一個row一個row填滿。。
- 可以加上其他**kwargs例如: facecolor, polar(default: False), projection(has to be a previously registered project)。
- 如果圖型會跨越兩個或以上個tile,可使用以下方式:
- 嘗試改為(222)(224)(121)。
- 若要有更複雜的子圖型配置,可以使用plt.GridSpec()方法。
- 首先使用plt.GridSpec(3,3,wspace=0.5,hspace=0.5)取得格網物件,3,3分別代表row與column個數,wspace與hspace則代表寬高兩向的圖型空隙。
- 在subplot()方法中直接使用grid[]來表示該子圖所占的位置,e.g. 0, 0:3為第0row,0,1,2column。
pyplot7.py
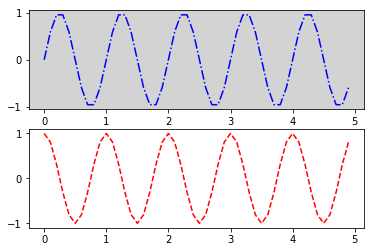
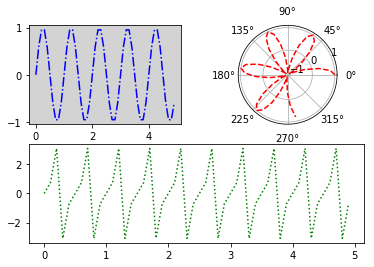
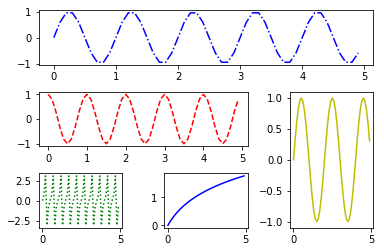
顯示圖片
- 顯示圖片的方式:
- 使用scipy.misc中的imread來讀取圖片。
- img2_tinted = img2*[1, 0.95, 0.9]: 圖型陣列成以顏色陣列(R,G,B),可修改顏色與色調。
- 使用imshow()方法來顯示圖片,使用np.unit8()方法來將其cast成unit8格式。
- 可使用plt.axis('off')來關閉x,y軸。
pyplot8.py
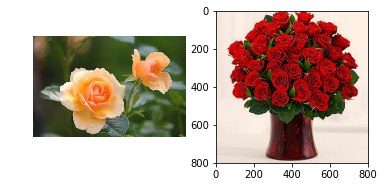